How to Install Mantine: A Step-by-Step Guide for Efficient Integration
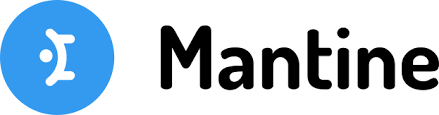
Mantine is a modern React component library that provides an extensive set of customizable UI components designed for faster, cleaner development. Whether you’re building a sleek dashboard or a full-blown application, Mantine’s focus on performance, accessibility, and ease of use makes it a great tool. If you’re new to Mantine, this article will help you understand how to install Mantine, configure it, and begin using its powerful features.
What Is Mantine?
Mantine is an open-source React component library designed to simplify and speed up the development of web applications. It includes over 100 easy-to-use and highly customizable components, enabling developers to build robust user interfaces quickly. It provides hooks, context API integration, and support for theming, making it flexible enough to fit into any project.
Why Use Mantine?
- Customizable Components: Mantine provides fully customizable UI elements such as modals, buttons, and sliders to meet your project’s branding and functionality requirements.
- Responsive Design: The components are designed with mobile-first responsiveness, ensuring a seamless user experience across devices.
- Developer-Friendly: Built with TypeScript support and modern JavaScript, Mantine enables faster development with less boilerplate code.
How to Install Mantine: Prerequisites
Before installing Mantine, you must ensure your development environment is set up correctly. Below are the prerequisites:
- Node.js and npm: Ensure you have the latest version of Node.js and npm installed. This is required to manage the dependencies of your project.
- React Project: Mantine is a React library, so you should have an existing React project or create a new one using the following command:
- bash
- Copy code
- npx create-react-app my-mantine-app
- cd my-machine-app
Now that the primary environment is ready, let’s install Mantine.
Step 1: Installing Mantine Core
The first step in understanding how to install Mantine is installing the core package. Mantine’s core library contains all the essential UI components you’ll need for your project. Follow the steps below:
- Open your terminal in the project directory.
- Run the following command:
- bash
- Copy code
- npm install @mantine/core @mantine/hooks
- This will install both the core components of Mantine and its hooks library.
Why Hooks?
Mantine’s hooks provide essential functions that simplify tasks such as form validation, state management, and more. For more flexibility, it’s highly recommended to install @mantine/hooks alongside the core package.
Step 2: Installing Mantine Provider for Styling
Mantine uses a centralized provider to control themes and apply global styling. To ensure Martine’s components work correctly with theming and other contextual needs, install the provider package:
bash
Copy code
npm install @mantine/styles
Once installed, wrap your application in the MantineProvider component. This will allow you to control the look and feel of your app globally.
Example:
js
Copy code
import React from ‘react’;
import ReactDOM from ‘react-dom’;
import { MantineProvider } from ‘@mantine/core’;
import App from ‘./App’;
ReactDOM.render(
<MantineProvider theme={{ color scheme: ‘light’ }}>
<App />
</MantineProvider>,
document.getElementById(‘root’)
);
Theme Customization
MantineProvider allows you to set themes (dark or light), spacing, and other global styles. You can adjust these parameters based on your project’s needs.
Step 3: Adding Essential Components
After installing Mantine’s core package and provider, you can build your UI. Let’s add standard components like buttons, text inputs, and a layout grid.
Example: Button Component
One of the most common UI elements is the button. Here’s how you can add a Mantine button component to your application:
js
Copy code
import { Button } from ‘@mantine/core’;
function App() {
return (
<div>
<Button variant=”outline”>Click me!</Button>
</div>
);
}
Mantine offers various button styles, including filled, outline, and gradient. You can customize the button’s color, size, and functionality to fit your project’s design.
Example: Grid Layout
To create responsive layouts, you can use the Mantine Grid component. Here’s a simple layout that adjusts based on screen size:
js
Copy code
import { Grid } from ‘@mantine/core’;
function LayoutExample() {
return (
<Grid>
< Grid.Col span={6}>Column 1</Grid.Col>
< Grid.Col span={6}>Column 2</Grid.Col>
</Grid>
);
}
Mantine’s Grid is similar to other popular CSS grid systems but has additional React-specific customization options, making it highly flexible.
Step 4: Installing Additional Packages (Optional)
Mantine offers additional packages for advanced functionalities. Depending on your project, you might need these extra packages. Here are some commonly used packages:
- @mantine/dates: Provides date pickers and calendars.
- bash
- Copy code
- npm install @mantine/dates
- @mantine/notifications: Adds notification components for alerts and messages.
- bash
- Copy code
- npm install @mantine/notifications
These packages integrate seamlessly with the core components and extend the functionality of your app.
Step 5: Form Handling with Mantine
Handling forms in Mantine is easy and intuitive. The user hook from Mantine allows you to manage form data and validation easily.
Example: Simple Form
js
Copy code
import { useForm } from ‘@mantine/hooks’;
import { TextInput, Button } from ‘@mantine/core’;
function SignupForm() {
const form = useForm({
initial values: {
email: ”,
},
validation rules: {
email: (value) => /^\S+@\S+$/.test(value),
},
});
return (
<form onSubmit={form.onSubmit((values) => console.log(values))}>
<TextInput
label=”Email”
placeholder=”[email protected]”
value={form. values.email}
onChange={(event) => form.setFieldValue(’email,’ event.currentTarget.value)}
error={form. Errors.email && ‘Invalid email’}
/>
<Button type=”submit”>Submit</Button>
</form>
);
}
In this example, the user hook manages the form’s state, validates the email field, and handles the form submission. You can extend this to include more form fields, custom validation rules, and other advanced features.
Step 6: Theming and Custom Styles
Using the MantineProvider, Customizing Mantine’s components is straightforward. You can define a custom theme, including colors, font sizes, and spacing.
Example: Custom Theme
js
Copy code
<MantineProvider
theme={{
colors: {
brand: [‘#f0f’, ‘#e0e’, ‘#d0d’, ‘#c0c’],
},
fontFamily: ‘Verdana, sans-serif’,
}}
>
<App />
</MantineProvider>
With this setup, you can control the overall appearance of your application while keeping it consistent across different components.
Final Thoughts
Mantine is an excellent UI library for React developers looking for flexibility and ease of use. Following the steps above, you’ll understand how to install Mantine and integrate it into your project efficiently. The library is well-documented and offers plenty of customization options, allowing you to build robust and visually appealing interfaces.
FAQs
- Can I use Mantine with TypeScript?
Yes, Mantine fully supports TypeScript. You can enjoy type safety across all components, making development faster and less error-prone.
- How do I customize Mantine components?
You can customize components through props, global theming, and CSS-in-JS solutions provided by Mantine.
- What are some alternatives to Mantine?
Some popular alternatives include Material-UI, Chakra UI, and Ant Design. However, Mantine offers a more modern approach with built-in hooks and a focus on accessibility.
- Is Mantine open source?
Yes, Mantine is an open-source project, and contributions are welcome via its GitHub repository.
By now, you should have a solid grasp of installing Mantine and using it effectively in your React projects. Mantine’s rich feature set and focus on customization make it an excellent choice for both small and large applications.
4o